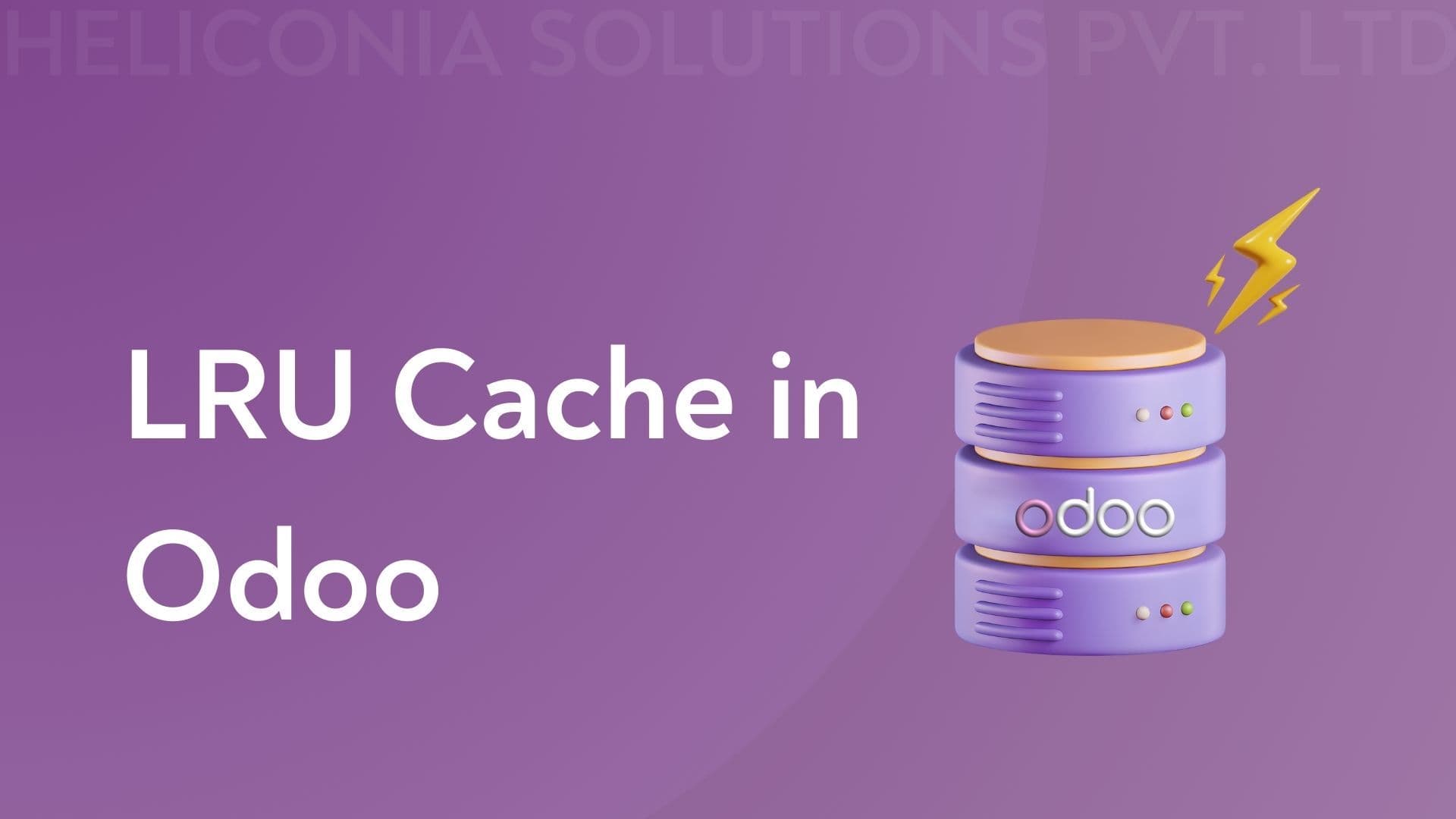
LRU Cache in Odoo: Optimizing Database Queries for Enhanced Performance
- Author: Atul Makwana
- Published: 8 months ago
- Category: Technical
- Time: 2 min read
Odoo utilizes an LRU (Least Recently Used) cache mechanism to improve performance within its system. This means Odoo prioritizes storing and retrieving data that has been accessed recently, while less frequently used data may be removed from the cache to make room for newer entries.
Benefits of LRU Cache in Odoo
Here's a breakdown of how LRU cache benefits Odoo:
- Faster loading times: Frequently accessed data is readily available in the cache, reducing the need to retrieve it from the main database every time. This leads to a smoother and quicker user experience.
- Improved performance: By caching frequently used data, Odoo minimizes the number of database queries it needs to perform, resulting in overall system efficiency.
- Reduced resource usage: By storing data in memory (cache) instead of constantly accessing the database, Odoo reduces the load on the server, saving resources.
When to Use LRU Cache in Odoo:
- Large Data Sets: LRU cache is beneficial in Odoo applications dealing with large data sets, such as product catalogs, customer records, or transactional data.
- Read-Heavy Workloads: Applications with read-heavy workloads, where the same data is accessed frequently, can benefit from LRU cache to optimize data retrieval and improve overall system performance.
- Computationally Intensive Operations: LRU cache can be used to cache the results of computationally intensive operations, such as complex business logic or data processing, to avoid redundant computations and speed up response times.
How to Implement LRU Cache in Odoo?
To implement an LRU (Least Recently Used) cache in Odoo, you can utilize Python's built-in functools.lru_cache
decorator. This decorator allows you to cache the results of a function based on its arguments, improving performance by avoiding repeated computation.
Here's how you can use functools.lru_cache
in Odoo:
- Import
lru_cache
: Import thelru_cache
decorator from thefunctools
module. - Decorate Your Function: Decorate the function that you want to cache with
@lru_cache
. - Specify Cache Parameters: You can specify the maximum size of the cache and other optional parameters such as
typed
,maxsize
, andtyped
.
Here's a simple example of using functools.lru_cache
in an Odoo module:
from odoo import api, models
from functools import lru_cache
class AccountMove(models.Model):
_name = 'account.move'
@api.model
@lru_cache(maxsize=128)
def search_products(self, company_id):
"""
Search all the products a company has access to.
This method is cached, only one search is done per company_id.
"""
return self.env['product.product'].search([
('company_id', 'in', (False, company_id))
])
let's understand the use of lru_cache
in the above code snippet
@lru_cache(maxsize=128)
: This decorator is imported from Python'sfunctools
module. It is used to cache the results of the function based on its input arguments. In this case, the results of thesearch_products
function will be cached based on thecompany_id
.maxsize=128
: This parameter specifies the maximum number of cached results to be stored. If set toNone
, the cache size is unlimited.- The primary purpose of using
@lru_cache
decorator here is to avoid redundant database queries for the samecompany_id
. Once the products for a specific company are searched for the first time, the results are cached. Subsequent calls tosearch_products
with the samecompany_id
will return the cached results instead of executing the search query again, thereby improving performance by reducing database load and query execution time.
In conclusion, the LRU cache serves as a hidden hero within Odoo, silently working behind the scenes to optimize performance. By prioritizing frequently accessed data, it ensures a faster and more responsive user experience. While you may not directly interact with the LRU cache, understanding its role empowers you to appreciate the intricate mechanisms that keep Odoo running smoothly.