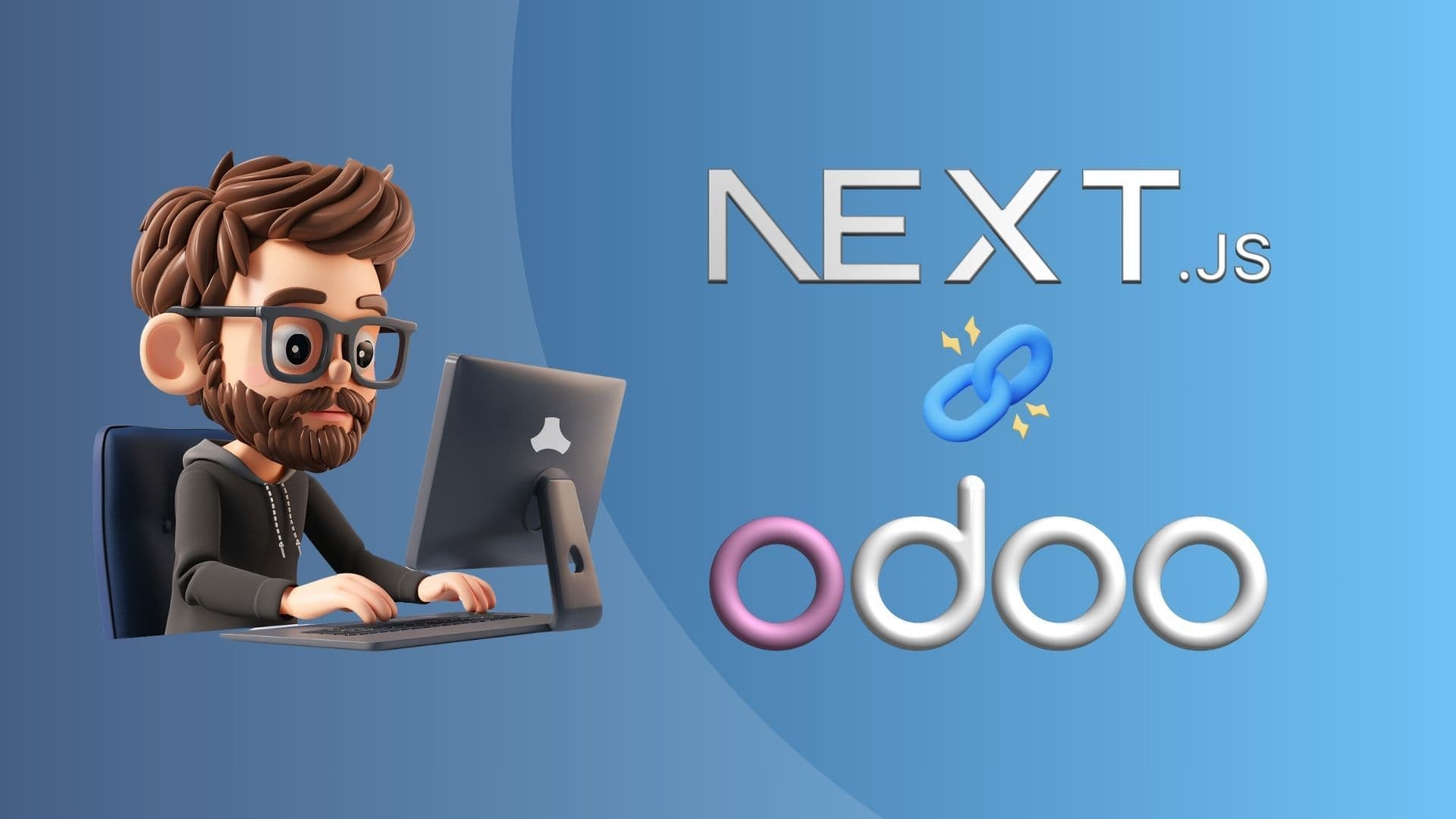
How to Integrate Next.js website with Odoo CRM?
- Author: Atul Makwana
- Published: 9 months ago
- Category: Odoo
- Time: 4 min read
Odoo, a powerful open-source ERP solution, excels in backend management, but its front end can feel restrictive. Enter Next.js, a modern React framework, offering a dynamic and customizable solution. This dream team – Next.js frontend and headless Odoo backend – creates a website powerhouse for lead generation and customer engagement.
Why Integrate Next.js with Odoo?
- Enhanced Performance: Next.js utilizes server-side rendering and automatic code-splitting, resulting in faster page loads and smoother user interactions compared to the traditional Odoo front-end.
- Improved SEO: With Next.js, developers have greater control over metadata and can optimize content for search engines, boosting visibility and driving organic traffic to your Odoo website.
- Flexibility and Customization: Next.js empowers developers to create highly customizable and responsive designs, allowing for a unique brand identity and tailored user experience.
- Modern Development Workflow: Leveraging React components and a streamlined development environment, Next.js offers a modern and efficient workflow for building and maintaining Odoo front-end projects.
In this comprehensive guide, we'll explore how to integrate your Next.js website's custom contact form with Odoo CRM leads, leveraging the power of Odoo Headless architecture. By seamlessly connecting Next.js with Odoo Headless, we'll outline a straightforward approach to capture leads from your website and synchronize them with your Odoo CRM system. With this method, you'll harness the endless possibilities of Next.js while efficiently managing your business contacts in Odoo CRM.
Step 1: Setting Up Your Next.js Project
First, make sure you have a Next.js project set up. If not, you can quickly create one using the following commands:
npx create-next-app@latest next-odoo
cd next-odoo
Step 2: Creating the Contact Form Component
Now, let's create a contact form component where users can input their information. create a `components` directory under src
(if you are not using src
directory you can create on the root of your project)
Now under the components
directory create a file ContactForm.tsx
if you do not want to use the typescript you can name it ContactForm.js
.
Here's an example of a basic contact form component in Next.js which you can add to your ContactForm.tsx
file :
import React, { useState } from 'react';
const ContactForm = () => {
const [formData, setFormData] = useState({
name: '',
email: '',
message: ''
});
const handleChange = (e) => {
setFormData({ ...formData, [e.target.name]: e.target.value });
};
const handleSubmit = async (e) => {
e.preventDefault();
// Call function to send form data to Odoo CRM
await sendFormDataToOdoo();
};
const sendFormDataToOdoo = async () => {
try {
const response = await fetch('/api/create-lead', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(formData)
});
if (response.ok) {
console.log('Lead created successfully in Odoo CRM!');
}
} catch (error) {
console.error('Error creating lead:', error);
}
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
name="name"
placeholder="Your Name"
value={formData.name}
onChange={handleChange}
/>
<input
type="email"
name="email"
placeholder="Your Email"
value={formData.email}
onChange={handleChange}
/>
<textarea
name="message"
placeholder="Your Message"
value={formData.message}
onChange={handleChange}
></textarea>
<button type="submit">Submit</button>
</form>
);
};
export default ContactForm;
Step 3: Setting Up Odoo API Endpoint
Next, we need to set up an API endpoint in our Next.js backend to send the form data to Odoo CRM. Create a new file called route.tsx
inside the app/api/create-lead
directory:
export async function POST(request: Request) {
const { name, email, message } = await request.json()
const response = await fetch(`${process.env.ODOO_API_URL}/api/create-lead`, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
// Add any necessary authentication headers here
},
body: JSON.stringify({ name, email, message })
});
if (response.ok) {
return Response.json({ success: true });
} else {
return Response.json({ success: false, error: 'Failed to create lead in Odoo CRM' });
}
}
in the above code snippet, you may notice the URL ${process.env.ODOO_API_URL}/api/create-lead
where you need to create the environment variable ODOO_API_URL
in .env
file which will hold the value of the url of your odoo instance.
ODOO_API_URL=https://www.your-odoo-instance.url
Step 4: Using Component in Contact Page
Now our component is ready we can use it on the contact page of the Next.js website. to do that we need to create a directory called contact
and inside it create a file called page.tsx
(use .js extension if you are using javascript).
import ContactForm from '@/components/ContactForm'
const ContactPage = () => {
return (
<>
<ContactForm/>
</>
)
}
export default ContactPage;
Step 5: Creating Odoo Controller to create CRM Lead
Odoo by default not provide the API to create the lead, so we need to create the API route to accept the request from the Next.js contact form. To do this we need to create a controller in the custom odoo module. (here we assume you already know how to create an odoo controller from a custom odoo module)
# controllers/main.py
from odoo import http
from odoo.http import request
import json
class LeadController(http.Controller):
@http.route('/api/create-lead', type='json', auth='public', methods=['POST'])
def create_lead(self, **kwargs):
try:
# Extract data from the request
name = kwargs.get('name')
email = kwargs.get('email')
message = kwargs.get('message')
# Create CRM lead
lead = request.env['crm.lead'].create({
'name': name,
'email_from': email,
'description': message,
# Add any other fields you want to populate
})
return {'success': True, 'lead_id': lead.id}
except Exception as e:
return {'success': False, 'error': str(e)}
Step 6: Testing the Next.js website integration with Odoo
To test the Next.js integration with Odoo CRM we need to run the Next.js using npm run dev
or yarn dev
. and also need to start the odoo instance, and make sure we install the custom addon that holds our LeadController.
now from the browser open the URL http//0.0.0.0:3000/contact
fill in the details of the contact form and hit submit. once you submit the form, it should create the crm.lead
record in the odoo. you can validate the record in the Odoo CRM pipeline
Congratulations!🎉 You've successfully integrated your Next.js website with Odoo CRM, allowing you to capture leads directly from your website and manage them seamlessly within your CRM system.
By following these steps, you can enhance your business operations and provide a smoother experience for your customers. If you have any questions or need further assistance, feel free to reach out to us at Heliconia Solutions. We're here to help you optimize your Next.js and Odoo integration for maximum efficiency and productivity.